Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Make iterator interface docs clearer (JuliaLang#50994)
A few beginner and intermediate Julia programmers shown both versions thought this one was clearer. Follows on from JuliaLang#50069 Two other minor changes: - includes `Base.isdone` in documenter output so that it can be linked from the table (this probably should have been done when we originally included it in the table). - update the warning on `isempty` about stateful iterators to be a little less strident, because `isempty` shouldn't cause any bugs for stateful iterators that correctly implement the documented interface (and I think I fixed all the stateful iterators in Base quite a while ago now). Before: 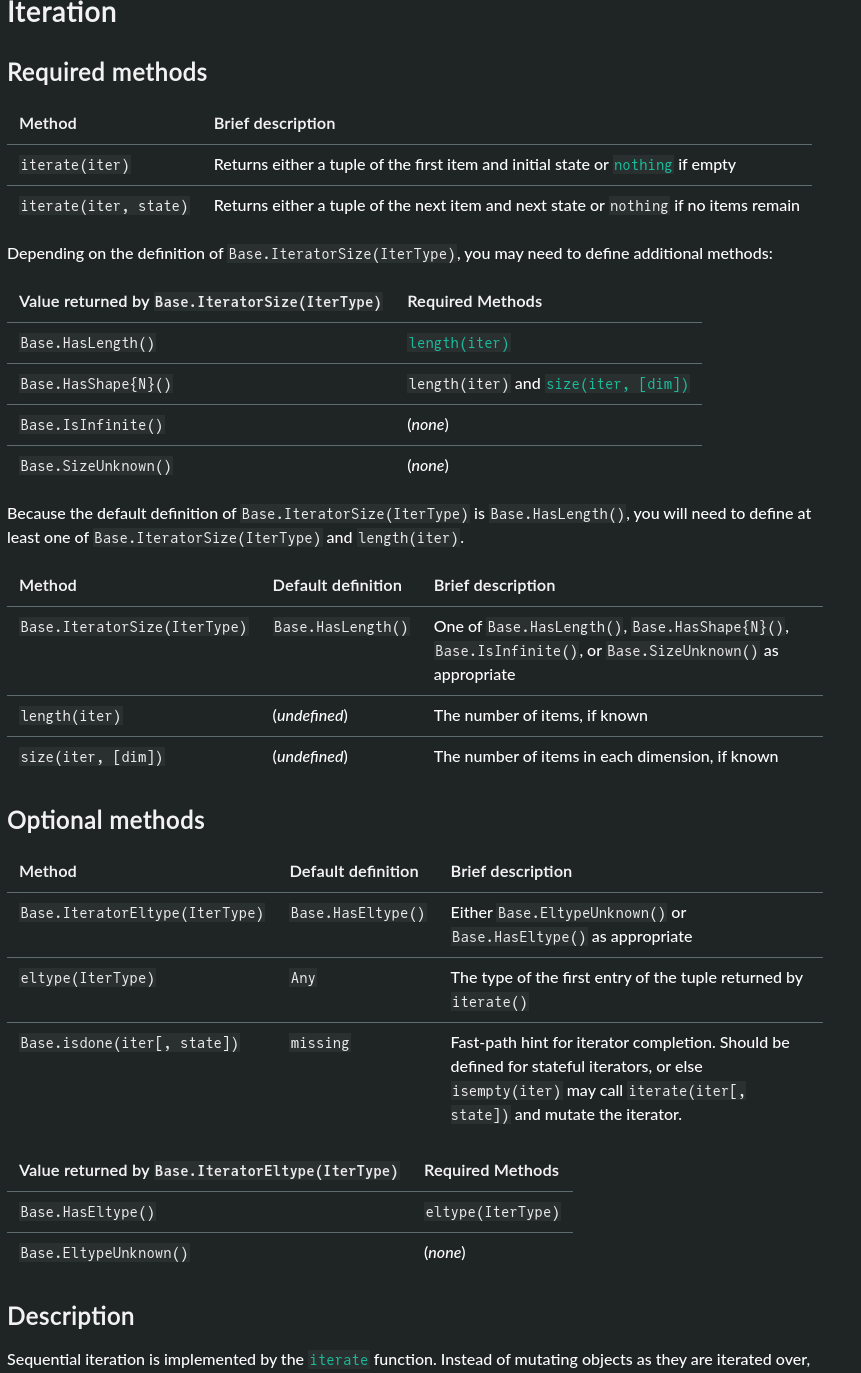 After:  I don't think it matters much, but the lengths of the columns are mostly determined by the longest `code` span within them, because the code spans are not wrapped. We can allow the browser to perform word wrapping within the spans by including `<wbr />` elements or unicode zero-width-space characters, but I can't get the cross references (links) to work. This is what I tried: ```md before [`Base.IteratorEltype(IterType)`](@ref) after (encouraging browsers to break the line at the dot): [<code>Base.<wbr />IteratorEltype(IterType)</code>](@ref Base.IteratorEltype) Which gives the following error: ┌ Error: reference for 'Base.IteratorEltype' could not be found in src/manual/interfaces.md. └ @ Documenter.CrossReferences ~/src/julia/doc/deps/packages/Documenter/yf96B/src/Utilities/Utilities.jl:32 ```
- Loading branch information