-
-
Notifications
You must be signed in to change notification settings - Fork 9.9k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Little Drag and Drop demo #1931
Comments
I now added an equivalent example in ShowDemoWindow(), with a little more options Also did some other work on drag and drop. However some things I couldn't do:
|
this is a reordering without move_from and move_to but with two extra data: first flag and dragdata
|
|
If the movement isn't reflected immediately then to be proper we should be displaying a target location in the form of a yellow LINE separating two items. This is a common pattern we'll also want to nicely resolve for tree nodes. |
Any plan to add this yellow LINE separating two items? Just tried implementing reorder today and found this issue. |
@zeyangl : I think adding Imgui::Separator as a drop target between elements can do it with some tweaks |
Jumping in as I'd love a yellow line when drag&drop separating two options for reordering... Please! |
I think some of it is discussed in #2823 but I am not sure what would be a good and lightweight API for it. |
LuaJIT code with a drag and drop demo
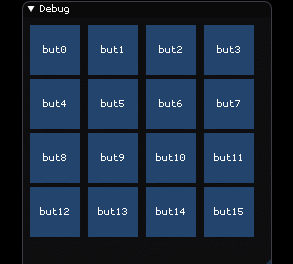
We have a butnum array
and use this code
The text was updated successfully, but these errors were encountered: