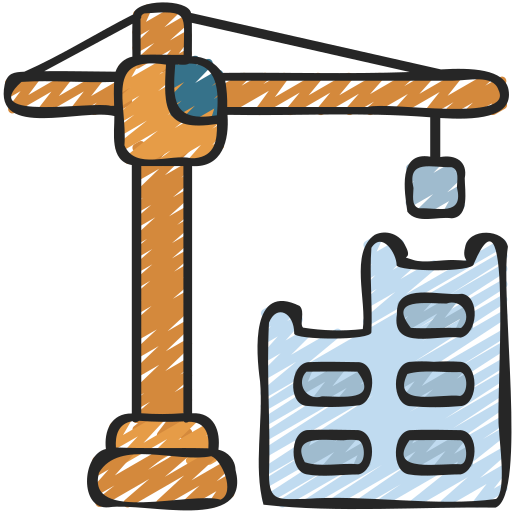
🏃 Getting Started | 📚 Documentation
Simple C# functions for building 🔨 requests and responses using only the core System.Net.Http!
Simple request response builders in C# without all the fancy frameworks.
- Just System.Net.Http
- Zero dependencies
- Easy to use and understand
- No additional allocations
- Does only what it says on the tin, builds requests and responses 🔨
using HttpBuildR;
// its helpful to alias this for readability
using Req = HttpMethod;
...
HttpRequestMessage request =
// start with the Http method
Req.Post
// now we can say where the request is made to
// any thing that is a string or Uri will work
// plays nice with the flurl Url builder!
.To("http:https://some-url/some-part")
// add some headers
.WithBearerToken(token)
.WithAccept("application/json")
.WithHeader("x-custom-header", "a","b","c")
// with some content, they are all supported!
.WithJsonContent(new {Name = "John", Age = 36});
// now you can send it!!!
...
To use this library, simply include HttpBuildR.Request.dll
in your project or
grab
it from NuGet, and add
this to the top of each .cs
file that needs it:
using HttpBuildR;
using Req = HttpMethod;
Note
If you're using implicit usings the above is not necessary.
Click through to the links bellow for further details.
Library | Description | Nu-Get |
---|---|---|
Request | Simple request builder functions on top of System.Net.Http! | |
Response | Simple response builder functions on top of System.Net.Http! | |
ActionResult | Simple ActionResult builder functions, building on-top of HttpBuildR.Response! |
There are a ton of http client libraries out there, but nothing (that I liked) that was just simple extensions to the core System.Net.Http classes.
I want the request and response building code to be,
- Declarative, it needs to flow and read as well as possible
- Simple, usage should be trivial and leaning curve as flat as possible
- Complete, anything you can do with the core class can be done in a fluent declarative style
For more details/information have a look the test projects or create an issue.