-
Notifications
You must be signed in to change notification settings - Fork 3.7k
Dragging Multiple Items in Sortable
Multi dragging is a powerful tool allowing users to select multiple items at a time within a Sortable list, and drag them as one item across multiple lists or within the same list. This page will go over the behavior of the multi-dragging functionality within SortableJS. It will also cover how to implement it into you project, and customize it to your project's requirements.
MultiDrag is a plugin for SortableJS, and is not included in all of Sortable's builds. It is available out of the box in the main Sortable.js file, but is not mounted by default in the modular builds (except for in sortable.complete.esm.js
).
To mount it from a modular build, simply follow this code:
import { Sortable, MultiDrag } from 'sortablejs';
Sortable.mount(new MultiDrag());
For multi-dragging to be possible, there must be a way for the user to select multiple items within the sortable before dragging begins. These items will all be dragged together once the user starts dragging any one of them. For an item to be selected, the user need only click it. If he wishes to deselect a selected item, clicking it again will deselect it.
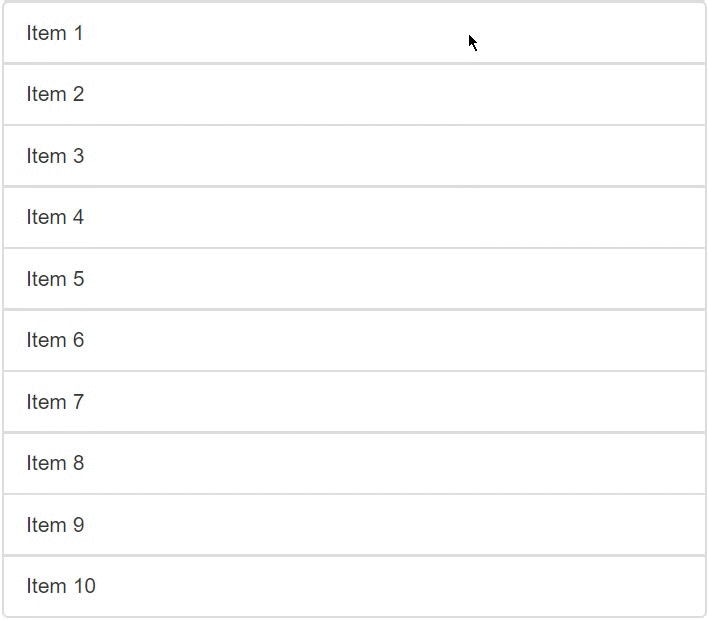
Sortable also allows for the user to select multiple items at a time. If the SHIFT
key is held down, and an item in the list is clicked, every item in the list from the last selected item to the clicked item will be selected. This emulates the SHIFT
key functionality in the "add-to-selection" function (selection with CTRL
key down) in UI systems such as the Windows File Explorer. If you wish to set a key that emulates the functionality of the "add-to-selection" key, use the multiDragKey
option.
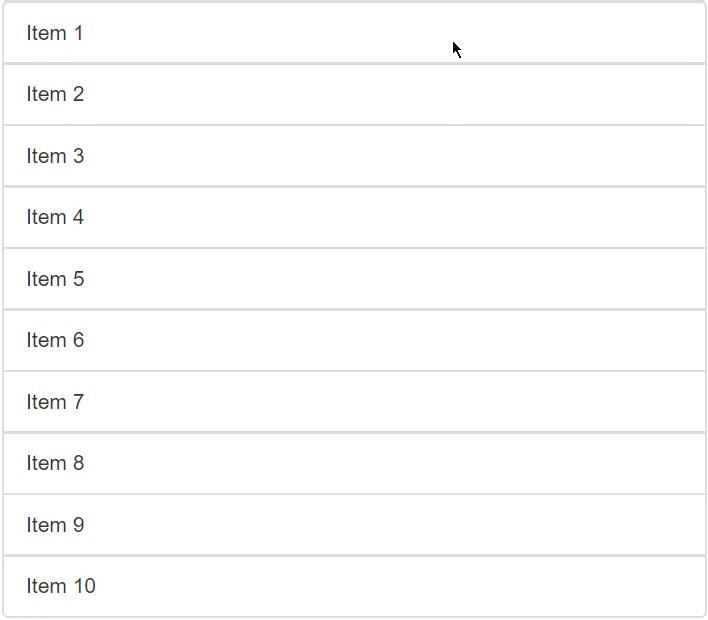
For the dragging of all the selected items to begin, the user must drag one of the selected items. All the selected items will then disappear (if animation is enabled, they will “fold” into the one selected item that the user is dragging) until the item is dropped.
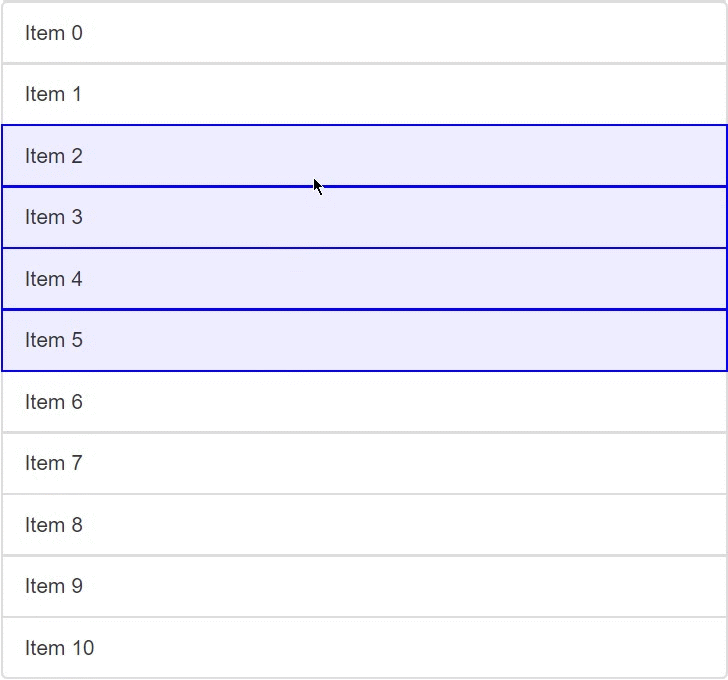
Lastly, when the item is dropped in a list, all the selected items that were dragged with it will be “unfolded” into their original orders beside each other at the position the item was dropped. Which specific item the user chose to drag will not affect the final order of the items.
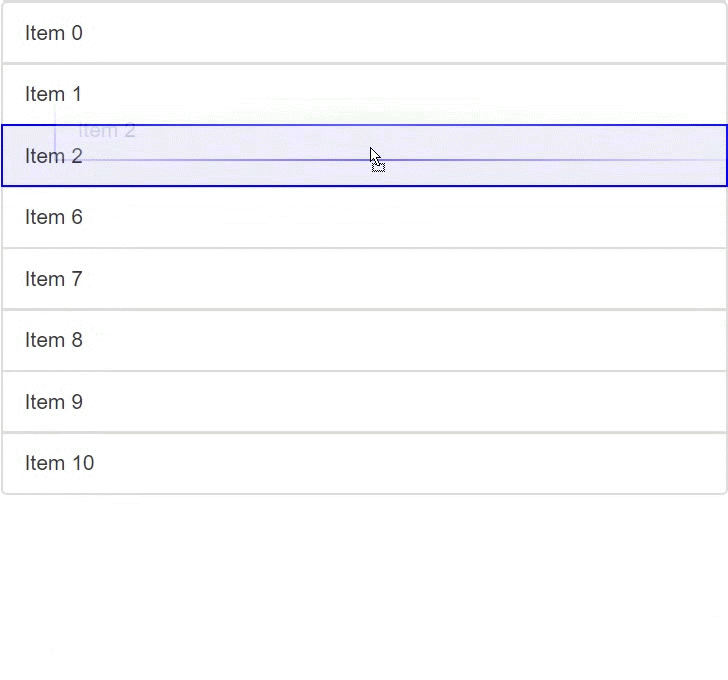
Enabling drag and drop is simple. After importing and mounting the plugin, all you must do is set multiDrag: true
in the options. However, it is impossible to use multi-dragging in production if a selectedClass
is not set (see below).
The selected class is the HTML class that will be applied to the selected elements in the list. Set this using the selectedClass
option.
A custom keyboard key may be designated as having to be down for the user to be able to select multi-drag elements. To set this key, use the multiDragKey
option. It is null
by default, meaning no key must be down.
The onSelect event is fired by Sortable when the user selects an item.
The onDeselect event is fired by Sortable when the user deselects an item.