Go is a popular programming language.
Go is used to create computer programs.
Go is a cross-platform, open source programming language
Go can be used to create high-performance applications
Go is a fast, statically typed, compiled language that feels like a dynamically typed, interpreted language
Go was developed at Google by Robert Griesemer, Rob Pike, and Ken Thompson in 2007
Go's syntax is similar to C++
Web development (server-side)
Developing network-based programs
Developing cross-platform enterprise applications
Cloud-native development
Concurrency is performing multiple things out-of-order, or at the same time, without affecting the final outcome
You can write more compact code, like shown below (this is not recommended because it makes the code more difficult to read):
package main
import ("fmt")
func main() {
fmt.Println("Hello World!")
}
Line 1: In Go, every program is part of a package. We define this using the package keyword. In this example, the program belongs to the main package.
Line 5: fmt.Println() is a function made available from the fmt package. It is used to output/print text. In our example it will output "Hello World!".
In Go, any executable code belongs to the main package.
You can also use comments to prevent the code from being executed.
The commented code can be saved for later reference and troubleshooting.
int- stores integers (whole numbers), such as 123 or -123
float32- stores floating point numbers, with decimals, such as 19.99 or -19.99
string - stores text, such as "Hello World". String values are surrounded by double quotes
bool- stores values with two states: true or false
Use the var keyword, followed by variable name and type:
Use the := sign, followed by the variable value:
Note: In this case, the type of the variable is inferred from the value (means that the compiler decides the type of the variable, based on the value).
Note: It is not possible to declare a variable using :=, without assigning a value to it.
If the value of a variable is known from the start, you can declare the variable and assign a value to it on one line:
In Go, all variables are initialized. So, if you declare a variable without an initial value, its value will be set to the default value of its type:
a
b
c
By running the code, we can see that they already have the default values of their respective types:
a is ""
b is 0
c is false
It is possible to assign a value to a variable after it is declared. This is helpful for cases the value is not initially known.
Note: It is not possible to declare a variable using ":=" without assigning a value to it.
This example shows declaring variables outside of a function, with the var keyword:
package main
import ("fmt")
func main() {
var a, b, c, d int = 1, 3, 5, 7
fmt.Println(a)
fmt.Println(b)
fmt.Println(c)
fmt.Println(d)
}
If the type keyword is not specified, you can declare different types of variables in the same line:
package main
import ("fmt")
func main() {
var a, b = 6, "Hello"
c, d := 7, "World!"
fmt.Println(a)
fmt.Println(b)
fmt.Println(c)
fmt.Println(d)
}
package main
import ("fmt")
func main() {
var (
a int
b int = 1
c string = "hello"
)
fmt.Println(a)
fmt.Println(b)
fmt.Println(c)
}
A variable can have a short name (like x and y) or a more descriptive name (age, price, carname, etc.).
A variable name must start with a letter or an underscore character (_)
A variable name cannot start with a digit
A variable name can only contain alpha-numeric characters and underscores (a-z, A-Z, 0-9, and _ )
Variable names are case-sensitive (age, Age and AGE are three different variables)
There is no limit on the length of the variable name
A variable name cannot contain spaces
The variable name cannot be any Go keywords
Variable names with more than one word can be difficult to read.
There are several techniques you can use to make them more readable:
Each word, except the first, starts with a capital letter:
myVariableName = "John"
Each word starts with a capital letter:
MyVariableName = "John"
Each word is separated by an underscore character:
my_variable_name = "John"
If a variable should have a fixed value that cannot be changed, you can use the const keyword.
The const keyword declares the variable as "constant", which means that it is unchangeable and read-only.
package main
import ("fmt")
const PI = 3.14
func main() {
fmt.Println(PI)
}
Constant names follow the same naming rules as variables
Constant names are usually written in uppercase letters (for easy identification and differentiation from variables)
Constants can be declared both inside and outside of a function
package main
import ("fmt")
const A int = 1
func main() {
fmt.Println(A)
}
package main
import ("fmt")
const A = 1
func main() {
fmt.Println(A)
}
Note: In this case, the type of the constant is inferred from the value (means the compiler decides the type of the constant, based on the value).
package main
import ("fmt")
const (
A int = 1
B = 3.14
C = "Hi!"
)
func main() {
fmt.Println(A)
fmt.Println(B)
fmt.Println(C)
}
Go has three functions to output text:
@ The Print() Function
The Print() function prints its arguments with their default format.
package main
import ("fmt")
func main() {
var i,j string = "Hello","World"
fmt.Print(i, "\n")
fmt.Print(j, "\n")
}
package main
import ("fmt")
func main() {
var i,j string = "Hello","World"
fmt.Print(i, "\n",j)
}
If we want to add a space between string arguments, we need to use " ":
we want to add a space between string arguments, we need to use " ":
package main
import ("fmt")
func main() {
var i,j string = "Hello","World"
fmt.Print(i, " ", j)
}
The Println() function is similar to Print() with the difference that a whitespace is added between the arguments, and a newline is added at the end:
package main
import ("fmt")
func main() {
var i,j string = "Hello","World"
fmt.Println(i,j)
}
The Printf() function first formats its argument based on the given formatting verb and then prints them.
package main
import ("fmt")
func main() {
var i string = "Hello"
var j int = 15
fmt.Printf("i has value: %v and type: %T\n", i, i)
fmt.Printf("j has value: %v and type: %T", j, j)
}
Go offers several formatting verbs that can be used with the Printf() function.
The following verbs can be used with all data types:
package main
import ("fmt")
func main() {
var i = 15.5
var txt = "Hello World!"
fmt.Printf("%v\n", i)
fmt.Printf("%#v\n", i)
fmt.Printf("%v%%\n", i)
fmt.Printf("%T\n", i)
fmt.Printf("%v\n", txt)
fmt.Printf("%#v\n", txt)
fmt.Printf("%T\n", txt)
}
package main
import ("fmt")
func main() {
var i = 15
fmt.Printf("%b\n", i)
fmt.Printf("%d\n", i)
fmt.Printf("%+d\n", i)
fmt.Printf("%o\n", i)
fmt.Printf("%O\n", i)
fmt.Printf("%x\n", i)
fmt.Printf("%X\n", i)
fmt.Printf("%#x\n", i)
fmt.Printf("%4d\n", i)
fmt.Printf("%-4d\n", i)
fmt.Printf("%04d\n", i)
}
`` golang package main import ("fmt")
func main() { var txt = "Hello"
fmt.Printf("%s\n", txt) fmt.Printf("%q\n", txt) fmt.Printf("%8s\n", txt) fmt.Printf("%-8s\n", txt) fmt.Printf("%x\n", txt) fmt.Printf("% x\n", txt) }
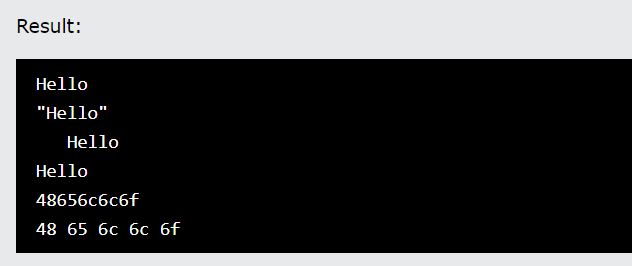
# Boolean Formatting Verbs
### The following verb can be used with the boolean data type:

``` golang
package main
import ("fmt")
func main() {
var i = true
var j = false
fmt.Printf("%t\n", i)
fmt.Printf("%t\n", j)
}
Data type is an important concept in programming. Data type specifies the size and type of variable values.
Go is statically typed, meaning that once a variable type is defined, it can only store data of that type.
package main
import ("fmt")
func main() {
var a bool = true // Boolean
var b int = 5 // Integer
var c float32 = 3.14 // Floating point number
var d string = "Hi!" // String
fmt.Println("Boolean: ", a)
fmt.Println("Integer: ", b)
fmt.Println("Float: ", c)
fmt.Println("String: ", d)
}
package main
import ("fmt")
func main() {
var b1 bool = true // typed declaration with initial value
var b2 = true // untyped declaration with initial value
var b3 bool // typed declaration without initial value
b4 := true // untyped declaration with initial value
fmt.Println(b1) // Returns true
fmt.Println(b2) // Returns true
fmt.Println(b3) // Returns false
fmt.Println(b4) // Returns true
}
Signed integers: can store both positive and negative values
Unsigned integers: - can only store non-negative values
Signed integers, declared with one of the int keywords, can store both positive and negative values:
package main
import ("fmt")
func main() {
var x uint = 500
var y uint = 4500
fmt.Printf("Type: %T, value: %v", x, x)
fmt.Printf("Type: %T, value: %v", y, y)
}
Arrays are used to store multiple values of the same type in a single variable, instead of declaring separate variables for each value.
Note: The length specifies the number of elements to store in the array. In Go, arrays have a fixed length. The length of the array is either defined by a number or is inferred (means that the compiler decides the length of the array, based on the number of values).
package main
import ("fmt")
func main() {
var arr1 = [3]int{1,2,3}
arr2 := [5]int{4,5,6,7,8}
fmt.Println(arr1)
fmt.Println(arr2)
}
package main
import ("fmt")
func main() {
var arr1 = [...]int{1,2,3}
arr2 := [...]int{4,5,6,7,8}
fmt.Println(arr1)
fmt.Println(arr2)
}
package main
import ("fmt")
func main() {
var cars = [4]string{"Volvo", "BMW", "Ford", "Mazda"}
fmt.Print(cars)
}
You can access a specific array element by referring to the index number.
In Go, array indexes start at 0. That means that [0] is the first element, [1] is the second element, etc.
This example shows how to access the first and third elements in the prices array:
package main
import ("fmt")
func main() {
prices := [3]int{10,20,30}
fmt.Println(prices[0])
fmt.Println(prices[2])
}
package main
import ("fmt")
func main() {
prices := [3]int{10,20,30}
prices[2] = 50
fmt.Println(prices)
}
package main
import ("fmt")
func main() {
prices := [3]int{10,20,30}
prices[2] = 50
fmt.Println(prices)
}
#Array Initialization
If an array or one of its elements has not been initialized in the code, it is assigned the default value of its type.
package main
import ("fmt")
func main() {
arr1 := [5]int{} //not initialized
arr2 := [5]int{1,2} //partially initialized
arr3 := [5]int{1,2,3,4,5} //fully initialized
fmt.Println(arr1)
fmt.Println(arr2)
fmt.Println(arr3)
}
package main
import ("fmt")
func main() {
arr1 := [5]int{1:10,2:40}
fmt.Println(arr1)
}
The array above has 5 elements.
1:10 means: assign 10 to array index 1 (second element).
2:40 means: assign 40 to array index 2 (third element).
package main
import ("fmt")
func main() {
arr1 := [4]string{"Volvo", "BMW", "Ford", "Mazda"}
arr2 := [...]int{1,2,3,4,5,6}
fmt.Println(len(arr1))
fmt.Println(len(arr2))
}
Slices are similar to arrays, but are more powerful and flexible.
Like arrays, slices are also used to store multiple values of the same type in a single variable.
However, unlike arrays, the length of a slice can grow and shrink as you see fit.
In Go, there are several ways to create a slice:
Using the []datatype{values} format
Create a slice from an array
Using the make() function
A common way of declaring a slice is like this:
The code above declares an empty slice of 0 length and 0 capacity.
To initialize the slice during declaration, use this:
The code above declares a slice of integers of length 3 and also the capacity of 3.
len() function - returns the length of the slice (the number of elements in the slice) cap() function - returns the capacity of the slice (the number of elements the slice can grow or shrink to)
package main
import ("fmt")
func main() {
myslice1 := []int{}
fmt.Println(len(myslice1))
fmt.Println(cap(myslice1))
fmt.Println(myslice1)
myslice2 := []string{"Go", "Slices", "Are", "Powerful"}
fmt.Println(len(myslice2))
fmt.Println(cap(myslice2))
fmt.Println(myslice2)
}
var myarray = [length]datatype{values} // An array
myslice := myarray[start:end] // A slice made from the array
package main
import ("fmt")
func main() {
arr1 := [6]int{10, 11, 12, 13, 14,15}
myslice := arr1[2:4]
fmt.Printf("myslice = %v\n", myslice)
fmt.Printf("length = %d\n", len(myslice))
fmt.Printf("capacity = %d\n", cap(myslice))
}
In the example above myslice is a slice with length 2. It is made from arr1 which is an array with length 6.
The slice starts from the second element of the array which has value 12. The slice can grow to the end of the array. This means that the capacity of the slice is 4.
If myslice started from element 0, the slice capacity would be 6.
slice_name := make([]type, length, capacity)
package main
import ("fmt")
func main() {
myslice1 := make([]int, 5, 10)
fmt.Printf("myslice1 = %v\n", myslice1)
fmt.Printf("length = %d\n", len(myslice1))
fmt.Printf("capacity = %d\n", cap(myslice1))
// with omitted capacity
myslice2 := make([]int, 5)
fmt.Printf("myslice2 = %v\n", myslice2)
fmt.Printf("length = %d\n", len(myslice2))
fmt.Printf("capacity = %d\n", cap(myslice2))
}
Access Elements of a Slice
You can access a specific slice element by referring to the index number.
In Go, indexes start at 0. That means that [0] is the first element, [1] is the second element, etc.
package main
import ("fmt")
func main() {
prices := []int{10,20,30}
fmt.Println(prices[0])
fmt.Println(prices[2])
}
You can also change a specific slice element by referring to the index number.
package main
import ("fmt")
func main() {
prices := []int{10,20,30}
prices[2] = 50
fmt.Println(prices[0])
fmt.Println(prices[2])
}
You can append elements to the end of a slice using the append()function:
package main
import (
"fmt"
)
func main() {
s := []int{1, 2, 3, 4, 5, 6}
fmt.Printf("s = %v\n", s)
fmt.Printf("length = %d\n", len(s))
fmt.Printf("capacity = %d\n", cap(s))
s = append(s, 20, 21, 22, 23, 24, 25)
fmt.Printf("s = %v\n", s)
fmt.Printf("length = %d\n", len(s))
fmt.Printf("capacity =%d\n", cap(s))
s = append(s, 30, 36)
fmt.Printf("s = %v\n", s)
fmt.Printf("length = %d\n", len(s))
fmt.Printf("capacity =%d\n", cap(s))
}
Conditional statements are used to perform different actions based on different conditions.
Go Conditions
A condition can be either true or false.
Go supports the usual comparison operators from mathematics:
Less than <
Less than or equal <=
Greater than >
Greater than or equal >=
Equal to ==
Not equal to !=
Additionally, Go supports the usual logical operators:
Logical AND &&
Logical OR ||
Logical NOT !
You can use these operators or their combinations to create conditions for different decisions.
package main
import ("fmt")
func main() {
x := 10
y := 5
fmt.Println(x > y)
}
package main
import ("fmt")
func main() {
x := 10
y := 5
fmt.Println(x != y)
}
package main
import ("fmt")
func main() {
x := 10
y := 5
z := 2
fmt.Println((x > y) && (y > z))
}
package main
import ("fmt")
func main() {
x := 10
y := 5
z := false
fmt.Println((x == y) || z)
}
Use if to specify a block of code to be executed, if a specified condition is true
Use else to specify a block of code to be executed, if the same condition is false
Use else if to specify a new condition to test, if the first condition is false
Use switch to specify many alternative blocks of code to be executed
Use the if statement to specify a block of Go code to be executed if a condition is true.
package main
import ("fmt")
func main() {
if 20 > 18 {
fmt.Println("20 is greater than 18")
}
}
package main
import ("fmt")
func main() {
x := 20
y := 18
if x>y {
fmt.Println("x is greater than y")
}
}
In the example above we use two variables, x and y, to test whether x is greater than y (using the > operator). As x is 20, and y is 18, and we know that 20 is
greater than 18, we print to the screen that "x is greater than y".
The else Statement
Use the else statement to specify a block of code to be executed if the condition is false.
In this example, time (20) is greater than 18, so the if condition is false. Because of this, we move on to the else condition and print to the screen "Good evening". If the time was less than 18, the program would print "Good day":
package main
import ("fmt")
func main() {
time := 20
if (time < 18) {
fmt.Println("Good day.")
} else {
fmt.Println("Good evening.")
}
}
In this example, the temperature is 14 so the condition for if is false so the code line inside the else statement is executed:
package main
import ("fmt")
func main() {
temperature := 14
if (temperature > 15) {
fmt.Println("It is warm out there")
} else {
fmt.Println("It is cold out there")
}
}
Use the else if statement to specify a new condition if the first condition is false.
package main
import ("fmt")
func main() {
time := 22
if time < 10 {
fmt.Println("Good morning.")
} else if time < 20 {
fmt.Println("Good day.")
} else {
fmt.Println("Good evening.")
}
}
In the example above, time (22) is greater than 10, so the first condition is false. The next condition, in the else if statement, is also false, so we move on to
else condition since condition1 and condition2 are both false - and print to the screen "Good evening".
However, if the time was 14, our program would print "Good day."
`` golang
package main
import ("fmt")
func main() { a := 14 b := 14 if a < b { fmt.Println("a is less than b.") } else if a > b { fmt.Println("a is more than b.") } else { fmt.Println("a and b are equal.") } }

## If conditions1 and condition2 are both correct, only the code for condition1 are executed:
``` golang
package main
import ("fmt")
func main() {
x := 30
if x >= 10 {
fmt.Println("x is larger than or equal to 10.")
} else if x > 20
fmt.Println("x is larger than 20.")
} else {
fmt.Println("x is less than 10.")
}
}
You can have if statements inside if statements, this is called a nested if.
package main
import ("fmt")
func main() {
num := 20
if num >= 10 {
fmt.Println("Num is more than 10.")
if num > 15 {
fmt.Println("Num is also more than 15.")
}
} else {
fmt.Println("Num is less than 10.")
}
}
Use the switch statement to select one of many code blocks to be executed.
The switch statement in Go is similar to the ones in C, C++, Java, JavaScript, and PHP. The difference is that it only runs the matched case so it does not need a
break statement.
The expression is evaluated once
The value of the switch expression is compared with the values of each case
If there is a match, the associated block of code is executed
The default keyword is optional. It specifies some code to run if there is no case match
package main
import ("fmt")
func main() {
day := 4
switch day {
case 1:
fmt.Println("Monday")
case 2:
fmt.Println("Tuesday")
case 3:
fmt.Println("Wednesday")
case 4:
fmt.Println("Thursday")
case 5:
fmt.Println("Friday")
case 6:
fmt.Println("Saturday")
case 7:
fmt.Println("Sunday")
}
}
The default keyword specifies some code to run if there is no case match:
package main
import ("fmt")
func main() {
day := 8
switch day {
case 1:
fmt.Println("Monday")
case 2:
fmt.Println("Tuesday")
case 3:
fmt.Println("Wednesday")
case 4:
fmt.Println("Thursday")
case 5:
fmt.Println("Friday")
case 6:
fmt.Println("Saturday")
case 7:
fmt.Println("Sunday")
default:
fmt.Println("Not a weekday")
}
}
All the case values should have the same type as the switch expression. Otherwise, the compiler will raise an error:
It is possible to have multiple values for each case in the switch statement:
The example below uses the weekday number to return different text:
package main
import ("fmt")
func main() {
day := 5
switch day {
case 1,3,5:
fmt.Println("Odd weekday")
case 2,4:
fmt.Println("Even weekday")
case 6,7:
fmt.Println("Weekend")
default:
fmt.Println("Invalid day of day number")
}
}
The for loop loops through a block of code a specified number of times.
The for loop is the only loop available in Go.
Loops are handy if you want to run the same code over and over again, each time with a different value.
Each execution of a loop is called an iteration.
The for loop can take up to three statements:
statement2 Evaluated for each loop iteration. If it evaluates to TRUE, the loop continues. If it evaluates to FALSE, the loop ends.
Note: These statements don't need to be present as loops arguments. However, they need to be present in the code in some form.
package main
func main() {
for i := 0; i < 5; i++ {
println(i)
}
}
i:=0; - Initialize the loop counter (i), and set the start value to 0
i < 5; - Continue the loop as long as i is less than 5
i++ - Increase the loop counter value by 1 for each iteration
package main
import ("fmt")
func main() {
for i:=0; i <= 100; i+=10 {
fmt.Println(i)
}
}
i:=0; - Initialize the loop counter (i), and set the start value to 0
i <= 100; - Continue the loop as long as i is less than or equal to 100
i+=10 - Increase the loop counter value by 10 for each iteration
The continue statement is used to skip one or more iterations in the loop. It then continues with the next iteration in the loop.
package main
import ("fmt")
func main() {
for i:=0; i < 5; i++ {
if i == 3 {
continue
}
fmt.Println(i)
}
}
The break statement is used to break/terminate the loop execution.
This example breaks out of the loop when i is equal to 3:
package main
import ("fmt")
func main() {
for i:=0; i < 5; i++ {
if i == 3 {
break
}
fmt.Println(i)
}
}
It is possible to place a loop inside another loop.
Here, the "inner loop" will be executed one time for each iteration of the "outer loop":
package main
import ("fmt")
func main() {
adj := [2]string{"big", "tasty"}
fruits := [3]string{"apple", "orange", "banana"}
for i:=0; i < len(adj); i++ {
for j:=0; j < len(fruits); j++ {
fmt.Println(adj[i],fruits[j])
}
}
}
The range keyword is used to more easily iterate over an array, slice or map. It returns both the index and the value.
The range keyword is used like this:
This example uses range to iterate over an array and print both the indexes and the values at each (idx stores the index, val stores the value):
package main
import ("fmt")
func main() {
fruits := [3]string{"apple", "orange", "banana"}
for idx, val := range fruits {
fmt.Printf("%v\t%v\n", idx, val)
}
}
package main
import ("fmt")
func main() {
fruits := [3]string{"apple", "orange", "banana"}
for _, val := range fruits {
fmt.Printf("%v\n", val)
}
}
Here, we want to omit the values (idx stores the index, val stores the value):
package main
import ("fmt")
func main() {
fruits := [3]string{"apple", "orange", "banana"}
for idx, _ := range fruits {
fmt.Printf("%v\n", idx)
}
A function is a block of statements that can be used repeatedly in a program.
A function will not execute automatically when a page loads.
A function will be executed by a call to the function.
To create (often referred to as declare) a function, do the following:
Specify a name for the function, followed by parentheses ().
Finally, add code that defines what the function should do, inside curly braces {}.
Functions are not executed immediately. They are "saved for later use", and will be executed when they are called.
In the example below, we create a function named "myMessage()". The opening curly brace ( { ) indicates the beginning of the function code, and the closing curly brace ( } ) indicates the end of the function. The function outputs "I just got executed!". To call the function, just write its name followed by two parentheses ():
package main
import ("fmt")
func myMessage() {
fmt.Println("I just got executed!")
}
func main() {
myMessage() // call the function
}
package main
import ("fmt")
func FunctionName() {
fmt.Println("I just got executed!")
}
func main() {
myMessage()
myMessage()
myMessage()
}
Information can be passed to functions as a parameter. Parameters act as variables inside the function.
Parameters and their types are specified after the function name, inside the parentheses. You can add as many parameters as you want, just separate them with a comma:
The following example has a function with one parameter (fname) of type string. When the familyName() function is called, we also pass along a name (e.g. Liam), and the name is used inside the function, which outputs several different first names, but an equal last name:
package main
import ("fmt")
func familyName(fname string) {
fmt.Println("Hello", fname, "Refsnes")
}
func main() {
familyName("Liam")
familyName("Jenny")
familyName("Anja")
}
Inside the function, you can add as many parameters as you want:
package main
import ("fmt")
func familyName(fname string, age int) {
fmt.Println("Hello", age, "year old", fname, "Refsnes")
}
func main() {
familyName("Liam", 3)
familyName("Jenny", 14)
familyName("Anja", 30)
}
Note: When you are working with multiple parameters, the function call must have the same number of arguments as there are parameters, and the arguments must be passed in the same order.
If you want the function to return a value, you need to define the data type of the return value (such as int, string, etc), and also use the return keyword inside the function:
Here, myFunction() receives two integers (x and y) and returns their addition (x + y) as integer (int):
package main
import ("fmt")
func myFunction(x int, y int) int {
return x + y
}
func main() {
fmt.Println(myFunction(1, 2))
}
In Go, you can name the return values of a function.
Here, we name the return value as result (of type int), and return the value with a naked return (means that we use the return statement without specifying the variable name):
package main
import ("fmt")
func myFunction(x int, y int) (result int) {
result = x + y
return
}
func main() {
fmt.Println(myFunction(1, 2))
}
The example above can also be written like this. Here, the return statement specifies the variable name:
package main
import ("fmt")
func myFunction(x int, y int) (result int) {
result = x + y
return result
}
func main() {
fmt.Println(myFunction(1, 2))
}
You can also store the return value in a variable, like this:
Here, we store the return value in a variable called total:
package main
import ("fmt")
func myFunction(x int, y int) (result int) {
result = x + y
return
}
func main() {
total := myFunction(1, 2)
fmt.Println(total)
}
Go functions can also return multiple values.
Here, myFunction() returns one integer (result) and one string (txt1):
package main
import ("fmt")
func myFunction(x int, y string) (result int, txt1 string) {
result = x + x
txt1 = y + " World!"
return
}
func main() {
fmt.Println(myFunction(5, "Hello"))
}
Here, we store the two return values into two variables (a and b):
package main
import ("fmt")
func myFunction(x int, y string) (result int, txt1 string) {
result = x + x
txt1 = y + " World!"
return
}
func main() {
a, b := myFunction(5, "Hello")
fmt.Println(a, b)
}
If we (for some reason) do not want to use some of the returned values, we can add an underscore (_), to omit this value.
Here, we want to omit the first returned value (result - which is stored in variable a):
package main
import ("fmt")
func myFunction(x int, y string) (result int, txt1 string) {
result = x + x
txt1 = y + " World!"
return
}
func main() {
_, b := myFunction(5, "Hello")
fmt.Println(b)
}
Here, we want to omit the second returned value (txt1 - which is stored in variable b):
package main
import ("fmt")
func myFunction(x int, y string) (result int, txt1 string) {
result = x + x
txt1 = y + " World!"
return
}
func main() {
a, _ := myFunction(5, "Hello")
fmt.Println(a)
}