versions now follow Angular's version to easily reflect compatibility.
Meaning, for Angular 10, use ngx-infinite-scroll @ ^10.0.0
Starting Angular 6 and Above - ngx-infinite-scroll@THE_VERSION.0.0
For Angular 4 and Angular = ^5.5.6 - use version [email protected]
For Angular 5.x with rxjs =<5.5.2 - use version [email protected]
For Angular version <= 2.3.1, you can use npm i angular2-infinite-scroll
(latest version is 0.3.42) - please notice the angular2-infinite-scroll package is deprecated
and much more.
These analytics are made available via the awesome Scarf package analytics library
Scarf can be disabled by following these directions
I'm a Senior Front End Engineer & Consultant at Orizens. My services include:
- Angular/React/Javascript Consulting
- Front End Architecture Consulting
- Project Code Review
- Project Development
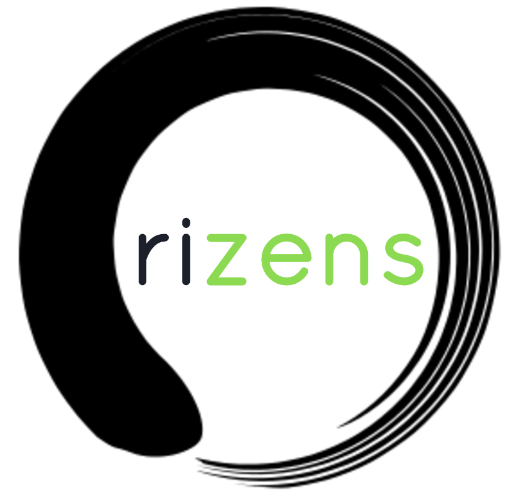
npm install ngx-infinite-scroll --save
@Input() | Type | Required | Default | Description |
---|---|---|---|---|
infiniteScrollDistance | number | optional | 2 | the bottom percentage point of the scroll nob relatively to the infinite-scroll container (i.e, 2 (2 * 10 = 20%) is event is triggered when 80% (100% - 20%) has been scrolled). if container.height is 900px, when the container is scrolled to or past the 720px, it will fire the scrolled event. |
infiniteScrollUpDistance | number | optional | 1.5 | should get a number |
infiniteScrollThrottle | number | optional | 150 | should get a number of milliseconds for throttle. The event will be triggered this many milliseconds after the user stops scrolling. |
scrollWindow | boolean | optional | true | listens to the window scroll instead of the actual element scroll. this allows to invoke a callback function in the scope of the element while listenning to the window scroll. |
immediateCheck | boolean | optional | false | invokes the handler immediately to check if a scroll event has been already triggred when the page has been loaded (i.e. - when you refresh a page that has been scrolled) |
infiniteScrollDisabled | boolean | optional | false | doesn't invoke the handler if set to true |
horizontal | boolean | optional | false | sets the scroll to listen for horizontal events |
alwaysCallback | boolean | optional | false | instructs the scroller to always trigger events |
infiniteScrollContainer | string / HTMLElement | optional | null | should get a html element or css selector for a scrollable element; window or current element will be used if this attribute is empty. |
fromRoot | boolean | optional | false | if infiniteScrollContainer is set, this instructs the scroller to query the container selector from the root of the document object. |
@Output() | Type | Event Type | Required | Description |
---|---|---|---|---|
scrolled | EventEmitter | IInfiniteScrollEvent | optional | this will callback if the distance threshold has been reached on a scroll down. |
scrolledUp | EventEmitter | IInfiniteScrollEvent | optional | this will callback if the distance threshold has been reached on a scroll up. |
By default, the directive listens to the window scroll event and invoked the callback.
To trigger the callback when the actual element is scrolled, these settings should be configured:
- [scrollWindow]="false"
- set an explict css "height" value to the element
First, import the InfiniteScrollModule to your module:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { InfiniteScrollModule } from 'ngx-infinite-scroll';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppComponent } from './app';
@NgModule({
imports: [BrowserModule, InfiniteScrollModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
platformBrowserDynamic().bootstrapModule(AppModule);
In this example, the onScroll callback will be invoked when the window is scrolled down:
import { Component } from '@angular/core';
@Component({
selector: 'app',
template: `
<div
class="search-results"
infiniteScroll
[infiniteScrollDistance]="2"
[infiniteScrollThrottle]="50"
(scrolled)="onScroll()"
></div>
`,
})
export class AppComponent {
onScroll() {
console.log('scrolled!!');
}
}
in this example, whenever the "search-results" is scrolled, the callback will be invoked:
import { Component } from '@angular/core';
@Component({
selector: 'app',
styles: [
`
.search-results {
height: 20rem;
overflow: scroll;
}
`,
],
template: `
<div
class="search-results"
infiniteScroll
[infiniteScrollDistance]="2"
[infiniteScrollThrottle]="50"
(scrolled)="onScroll()"
[scrollWindow]="false"
></div>
`,
})
export class AppComponent {
onScroll() {
console.log('scrolled!!');
}
}
In this example, the onScrollDown callback will be invoked when the window is scrolled down and the onScrollUp callback will be invoked when the window is scrolled up:
import { Component } from '@angular/core';
import { InfiniteScroll } from 'ngx-infinite-scroll';
@Component({
selector: 'app',
directives: [InfiniteScroll],
template: `
<div
class="search-results"
infiniteScroll
[infiniteScrollDistance]="2"
[infiniteScrollUpDistance]="1.5"
[infiniteScrollThrottle]="50"
(scrolled)="onScrollDown()"
(scrolledUp)="onScrollUp()"
></div>
`,
})
export class AppComponent {
onScrollDown() {
console.log('scrolled down!!');
}
onScrollUp() {
console.log('scrolled up!!');
}
}
In this example, the infiniteScrollContainer attribute is used to point directive to the scrollable container using a css selector. fromRoot is used to determine whether the scroll container has to be searched within the whole document ([fromRoot]="true"
) or just inside the infiniteScroll directive ([fromRoot]="false"
, default option).
import { Component } from '@angular/core';
@Component({
selector: 'app',
styles: [
`
.main-panel {
height: 100px;
overflow-y: scroll;
}
`,
],
template: `
<div class="main-panel">
<div
infiniteScroll
[infiniteScrollDistance]="2"
[infiniteScrollThrottle]="50"
[infiniteScrollContainer]="selector"
[fromRoot]="true"
(scrolled)="onScroll()"
></div>
</div>
`,
})
export class AppComponent {
selector: string = '.main-panel';
onScroll() {
console.log('scrolled!!');
}
}
It is also possible to use infiniteScrollContainer without additional variable by using single quotes inside double quotes:
[infiniteScrollContainer]="'.main-panel'"
This project exists thanks to all the people who contribute. [Contribute].
Thank you to all our backers! 🙏 [Become a backer]