# Swift Client for Free Ton SDK
[](https://swift.org/package-manager/)
Swift is a strongly typed language that has long been used not only for iOS development. Apple is actively promoting it to new platforms and today it can be used for almost any task. Thanks to this, this implementation provides the work of TonSDK on many platforms at once, including the native one for mobile phones. Let me remind you that swift can also be built for android.
| OS | Result |
| ----------- | ----------- |
| MacOS | ✅ |
| Linux | ✅ |
| iOS | ✅ |
| Windows | Soon |
## Usage
All requests are async
```swift
import TonClientSwift
var config: TSDKClientConfig = .init()
config.network = TSDKNetworkConfig(server_address: "https://net.ton.dev")
let client: TSDKClientModule = .init(config: config)
// Crypto
client.crypto.factorize(TSDKParamsOfFactorize(composite: "17ED48941A08F981")) { (response) in
print(response.result?.factors)
}
// Boc
let payload: TSDKParamsOfParse = .init(bocEncodedBase64: "te6ccgEBAQEAWAAAq2n+AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAE/zMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzSsG8DgAAAAAjuOu9NAL7BxYpA")
client.boc.parse_message(payload) { (response) in
if let result = response.result, let parsed: [String: Any] = result.parsed.toDictionary() {
print(parsed["id"])
print(parsed["src"])
print(parsed["dst"])
}
}
```
## Errors
```swift
client.crypto.factorize(TSDKParamsOfFactorize(composite: "17ED48941A08F981")) { (response) in
if let error = response.error {
print(error.data.toJSON())
print(error.code)
}
}
```
## Setup TONSDK For Linux and MacOS
### Install sdk with bash script
0. ```cd path_to_this_library```
1. ```bash scripts/install_tonsdk.sh```
### Manual install sdk ( if you have any problem with script install_tonsdk.sh )
SPOILER: Manual installation
0. Install Rust to your OS
1. git clone https://github.com/tonlabs/TON-SDK
2. cd ./TON-SDK
3. cargo update
4. cargo build --release
5. copy or create symlink of dynamic library
__macOS :__
**./TON-SDK/target/release/libton_client.dylib**
to
**/usr/local/lib/libton_client.dylib**
__Linux :__
**./TON-SDK/target/release/libton_client.so**
to
**/usr/lib/libton_client.so**
6. Create pkgConfig file :
__macOS :__
**/usr/local/lib/pkgconfig/libton_client.pc**
```bash
prefix=/usr/local
exec_prefix=${prefix}
includedir=${prefix}/include
libdir=${exec_prefix}/lib
Name: ton_client
Description: ton_client
Version: 1.0.0
Cflags: -I${includedir}
Libs: -L${libdir} -lton_client
```
__Linux:__
**/usr/lib/pkgconfig/libton_client.pc**
```bash
prefix=/usr
exec_prefix=${prefix}
includedir=${prefix}/include
libdir=${exec_prefix}/lib
Name: ton_client
Description: ton_client
Version: 1.0.0
Cflags: -I${includedir}
Libs: -L${libdir} -lton_client
```
7. Copy or create symlink of file
**/TON-SDK/ton_client/client/tonclient.h**
to
__MacOS:__
**/usr/local/include/tonclient.h**
__Linux:__
**/usr/include/tonclient.h**
## Setup TONSDK For iOS
0. For install Rust and rust dependencies you should download and execute the install_rust.sh script from scripts directory
```bash install_rust.sh```
⚠️ Wait installation
1.
```
git clone https://github.com/tonlabs/TON-SDK.git
cd ./TON-SDK
cargo lipo --release
```
⚠️ Wait installation
2. In xcode __File > Add files to "Name Your Project"__ navigate to ./TON-SDK/ton_client/tonclient.h
3. Create bridge. In xcode __File > New > File__, select Header File, set name for example Tonclient-Bridging-Header.h and add __#include __ and __#import "tonclient.h"__ like this:
```C
#ifndef Tonclient_Bridging_Header_h
#define Tonclient_Bridging_Header_h
#include
#import "tonclient.h"
#endif
```
4. Add path to search for bridge headers ( path to Tonclient-Bridging-Header.h )
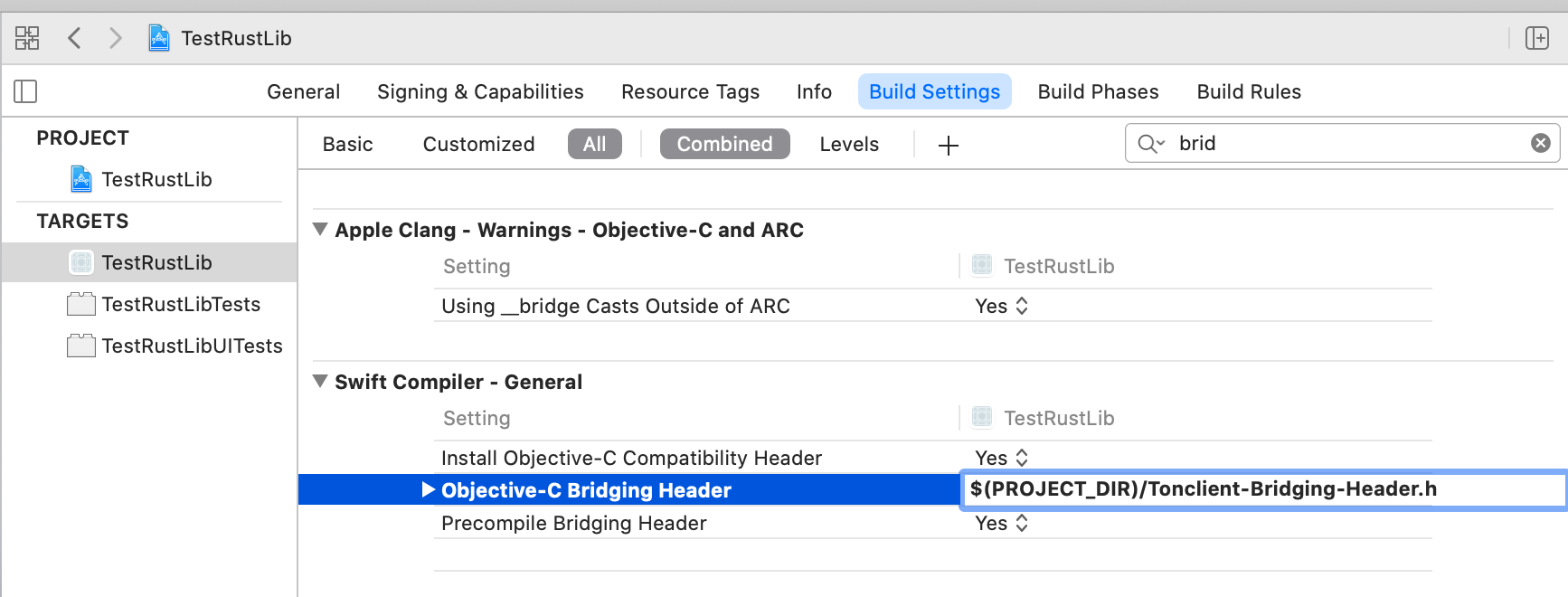
5. Add path to search for libraries ( path to directory withlibton_client.a )
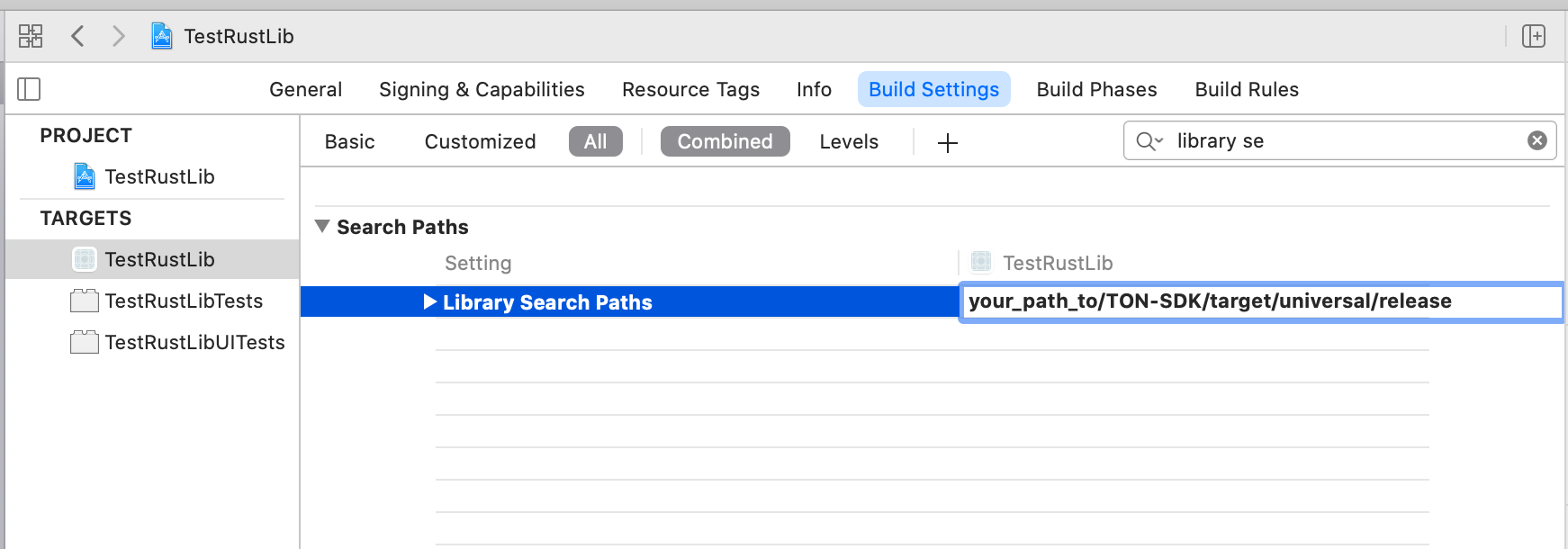
6. __File > Swift Packages > Add Package Dependency__
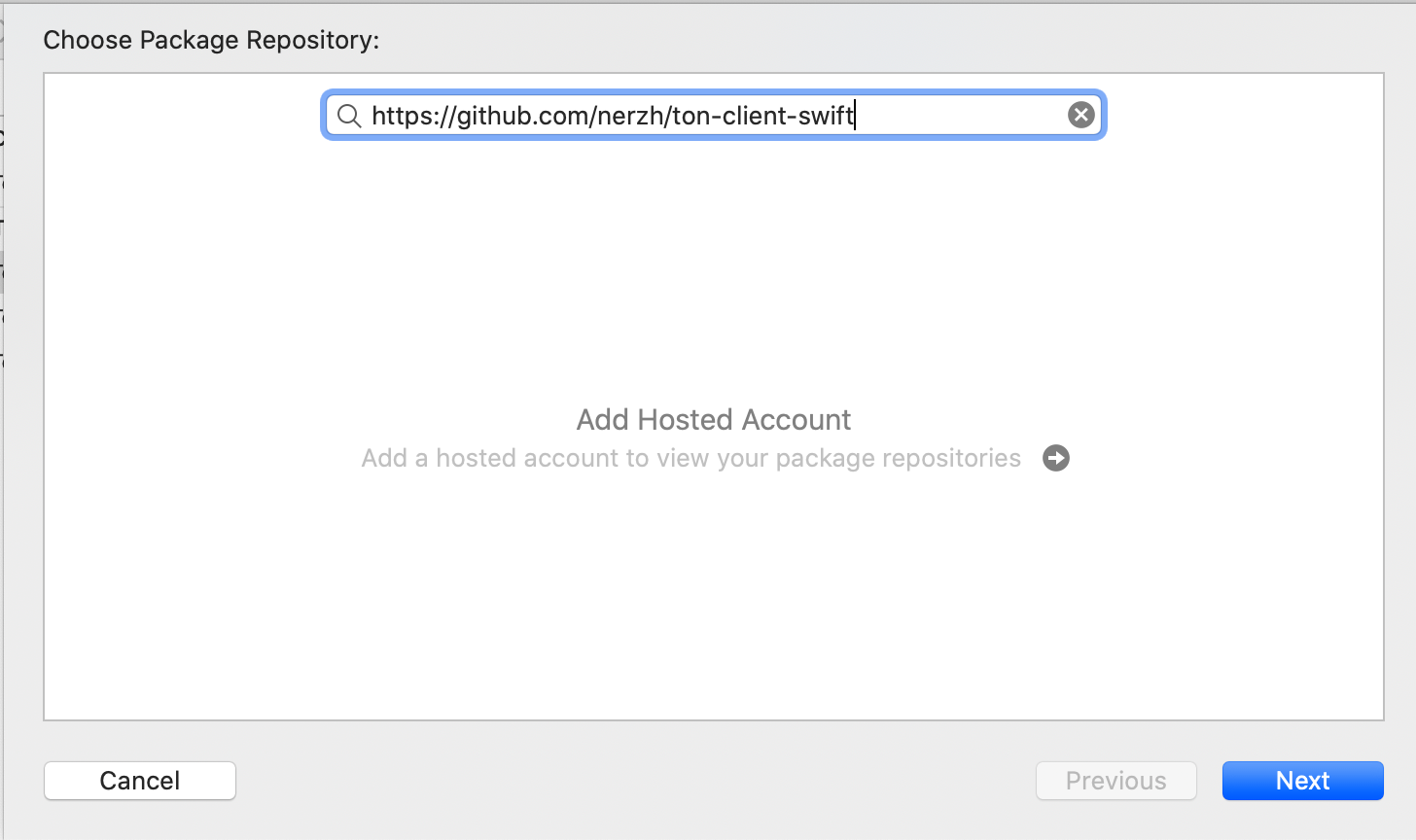
7. Build project ...
## Tests
### If you use Xcode for Test
Please, set custom working directory to project folder for your xcode scheme. This is necessary for the relative path "./" to this library folders.
You may change it with the xcode edit scheme menu __Product > Scheme > Edit Scheme__ menu __Run__ submenu __Options__ enable checkbox "Use custom directory" and add custom working directory.
Or if above variant not available, then inside file path_to_this_library/.swiftpm/xcode/xcshareddata/xcschemes/TonClientSwift.xcscheme
set to tag __"LaunchAction"__ absolute path to this library with options:
**useCustomWorkingDirectory = "YES"**
**customWorkingDirectory = "/path_to_this_library"**
### Tests
1. inside root directory of ton-client-swift create **.env.debug** file with
NET TON DEV
```
server_address=https://net.ton.dev
giver_address=0:653b9a6452c7a982c6dc92b2da9eba832ade1c467699ebb3b43dca6d77b780dd
giver_abi_name=Giver
giver_function=grant
```
**Optional:** Install locale NodeSE for tests if you needed:
- launch docker
- docker run -d --name local-node -p 80:80 tonlabs/local-node
nodeSE will start on localhost:80
```
server_address=http://localhost:80
giver_address=0:841288ed3b55d9cdafa806807f02a0ae0c169aa5edfe88a789a6482429756a94
giver_abi_name=GiverNodeSE
giver_function=sendGrams
giver_amount=10000000000
```
2. Run Tests
__MacOS:__
Run Tests inside Xcode
__Linux:__
swift test --generate-linuxmain
swift test --enable-test-discovery