You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Currently streaming 4RX channels with a N310 (GNSS reflectometry application). I can record and then navigate with the produced IF data files. When analysing these files and creating histrograms I've noticed that the samples are only occupying a small part of the SC16 sample bit depth. Shown by the following image (histograms for I and Q):
I've tried sampling a CW tone at -20dBm and the histogram is also limited to only +-40 compared to the full +-32767 of a signed short. Clearly this is saturating the ADC but why is it bounded by 40?
Interested to see whether anyone else can replicate this behaviour. I've attached my code below. Let me know if I'm doing something silly. I have a hunch this is why I'm receiving low C/No values when navigating with the produced baseband IF files.
Issue Description
Currently streaming 4RX channels with a N310 (GNSS reflectometry application). I can record and then navigate with the produced IF data files. When analysing these files and creating histrograms I've noticed that the samples are only occupying a small part of the SC16 sample bit depth. Shown by the following image (histograms for I and Q):
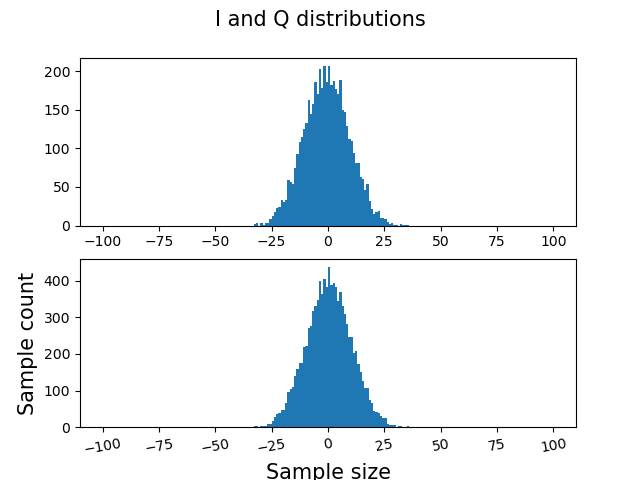
I've tried sampling a CW tone at -20dBm and the histogram is also limited to only +-40 compared to the full +-32767 of a signed short. Clearly this is saturating the ADC but why is it bounded by 40?
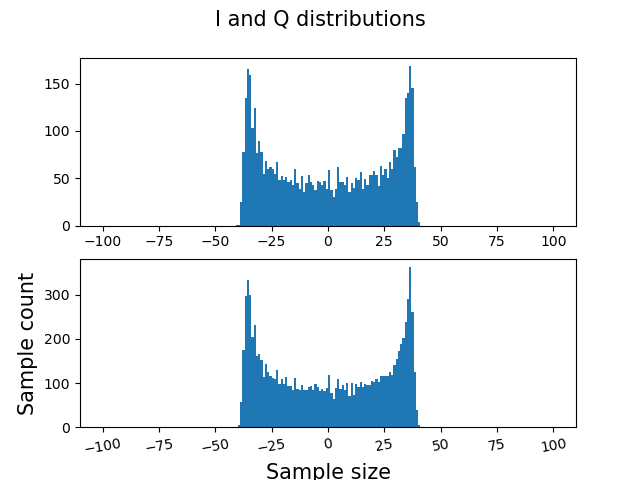
Interested to see whether anyone else can replicate this behaviour. I've attached my code below. Let me know if I'm doing something silly. I have a hunch this is why I'm receiving low C/No values when navigating with the produced baseband IF files.
Setup Details
The text was updated successfully, but these errors were encountered: